Week 1: Praat basics
1 The basics
Praat is one of the best tools available for research with speech sounds, and even though the interface might not look incredibly user-friendly, it is indeed a quite versatile program, especially when you can write your own scripts. We will become familiar with the wide range of possibilities that Praat offers as we go.
If you already have experience with programming, you might recall that Hello world is always the first assignment. After opening Praat, we go to the menu and choose Praat >> New Praat script. A window called Untitled script will appear.
1.1 Writing to the Info Window: Hello world
We type down the following (do not type the number 1; that is just showing you the line number! And mind the capitals too).
writeInfoLine: "Hello world!"
And then we go to the menu on the script window and hit Run >> Run. This is pretty much all you need in order to get some text printed. However, make sure that these texts are always in quotation marks.
Now, right below the previous line, type the following and then hit Run:
writeInfoLine: "Yikes, I deleted the previous line!"
You will see that ”Hello world” will disappear. So, now we will use appendInfoLine instead of writeInfoLine. See what happens.
appendInfoLine: "I will not delete the line above"
You may also use the operator + to concatenate text:
writeInfoLine: "Hello, " + "I am a Praat line"
Or you can also use a comma:
writeInfoLine: "Hello, " , "I am a Praat line"
1.3 String variables
Scripting often requires to store strings of text as variables; for instance, a file name. More importantly, we will see that these string variables will be very useful in for loops; we will talk more about it next week. So, we can store a string in Praat's short term memory by creating a string variable. The syntax is: nameofyourvariable$ = "String of text". You may name this variable in whatever way you want, as long as (a) You don't start with a capital letter; and (b) you add the dollar sign at the end of it. Here are examples:
first$= "Praat scripting"
second$= "makes things easier"
Importantly, we can do things with these variables. For instance, we can make them be part of a certain text. Type the script below and hit Run when ready.
myname$="Fernanda"
string1$= "Praat"
string2$= "makes things easier"
writeInfoLine: "I am not entirely sure whether " , string1$, " ", string2$
appendInfoLine: "Maybe it's not ", string1$
appendInfoLine: "It's " , myname$, " who ", string2$
Remember that the dollar sign is how we tell Praat that myname$, string1$, string2$ are string variables and not commands or an undefined numeric variable. We will use string variables for opening files in section 2.
1.2 Numeric variables
We can also create numeric variables; that is, we store a given number under a name. This is a very frequent procedure in Praat. We will write a small script that shows the total number of letters in your name. We create the variable firstname and we put the number of letters in your first name. Then we create lastname. Note: you can also call these variables whatever way you want, as long as they don't start with a capital letter.
firstname= 8
lastname=10
writeInfoLine: "My name has ", firstname + lastname , " letters in total"
So this way we have asked the computer to store a number under a certain name, so that we can invoke this value later by using this name instead of typing the number again. Note that numeric variables do not go in quotation marks and that their names do not end with a dollar sign.
Note also that Praat remembers only the variables that are within the script you're running.
Quick exercise: Which of these are "legal" variable assignments/operations? If you're unsure open a new script, try to do the operations and see if Praat complains. If it does, correct the mistakes!
soundfile$ = "recording.wav"
age = 28
age$ = "28"
sound file$ = "recording.wav"
Dir$ = "/home/User/Desktop/praat scripting/"
dir$ = "/home/User/Desktop/praat_scripting/"
filepath$ = dir$ + soundfile$
2timesAge = 2 * age
agePlusAge$ = age$ + age$
2 A small example: open and play file number 3
In Praat you can do things two different ways: one is to click on the buttons that you see in the Objects window, and the other one is by scripting. However, what a script does is to basically replace your clicks with lines of code; the code is still telling Praat to "click" on the options.
In order to illustrate this point, we will play with a little bunch of words in Spanish. You can download them from this link. Unzip the file and put the folder on the Desktop.
Now we will open the files the old fashioned way (by clicking). Go to Open >> Read from file... and select the files that you want to open. That's pretty much it, and sometimes it may be more useful than typing a script, but if you work with lots of files and something in your scripts is not working properly, you might not be so inclined to open the files manually everytime your scripts give you error messages (and this will happen a lot, unfortunately).
Now, type down this script. Pay attention to line 1: you will see that we are defining a string variable with the path to the folder on my computer. Here you need to type down the path to the palabras folder on your computer. If you actually have the palabras folder on the desktop, and if you are on a Windows computer, a path would look something like this: C:/Users/username/Desktop/palabras/; on a Mac, it would be something like /Users/username/Desktop/palabras/, and on Linux, something like /home/username/Desktop/palabras/. The rest of the script can be left as is. Hit Run when ready.
sound_directory$ = "/home/fernanda/Desktop/palabras/"
Create Strings as file list: "fileList", sound_directory$ + "*.wav"
anumber = 3
thisfile$ = Get string: anumber
Read from file: sound_directory$ + thisfile$
Play
Let's analyze what happened here. But first, look at the script and try to figure out which line does what. The answers are after this panda picture.
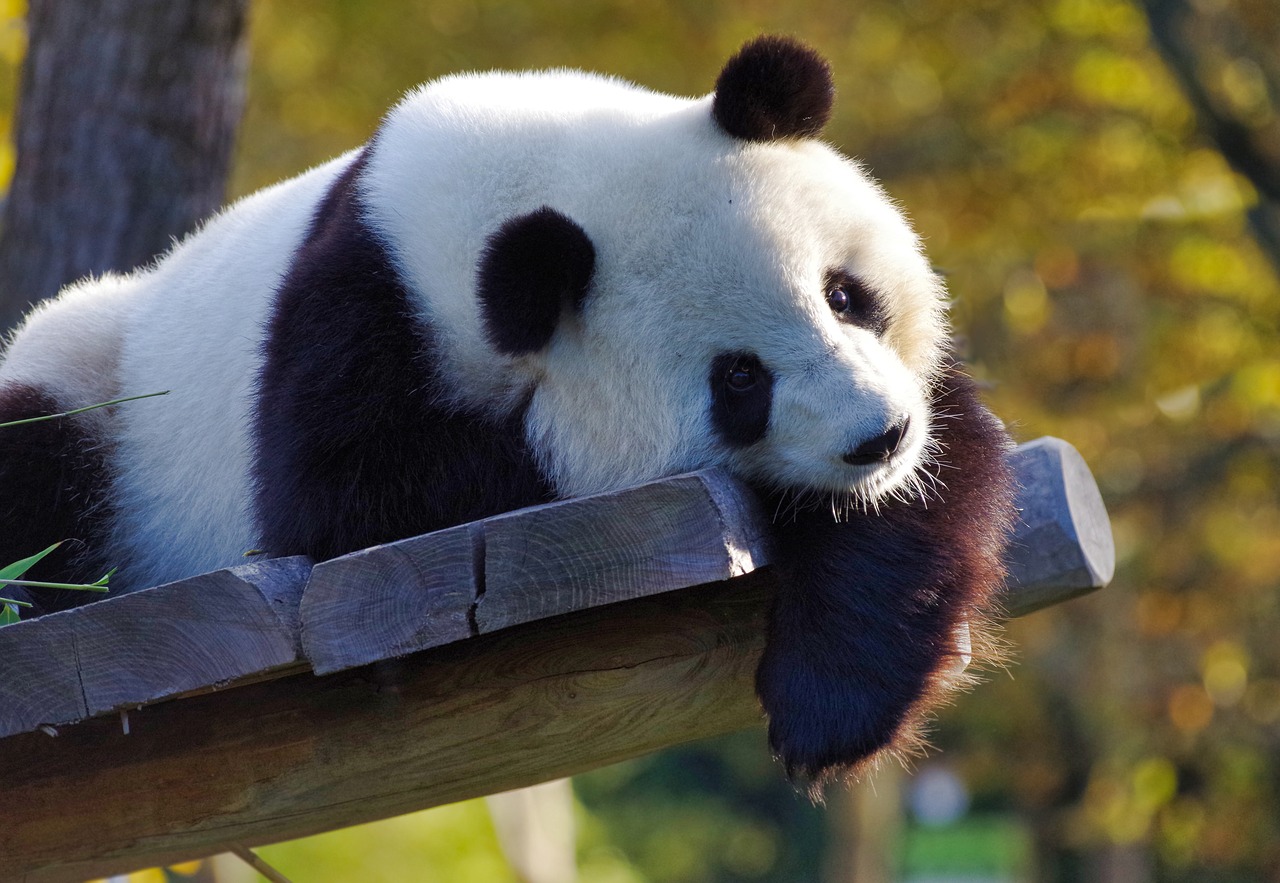
Line 1 creates a string variable called sound_directory$, which contains the path to the folder palabras. Line 2 creates a Strings list (creatively called "fileList"; this is the first argument of the Create Strings as file list command). Strings lists are one of the many objects you can create with Praat (we will see throughout the course that Strings lists can be very useful). The second argument of this command is the path to the folder, which is stored as the string variable sound_directory$. After that comes a "*.wav" string, which means that we want Praat to open all the files with the .wav extension (the asterisk * is a wildcard).
You can also create a strings list by clicking. In order to do so, go to the Objects window and go to New >> Strings >> Create Strings as file list. You will see that this window has two text fields, which correspond to the arguments that we passed to the Create Strings as file list command in the script.
Now, you will see that there is a new Object in the Objects window, called Strings fileList. If you open it, you will see that it's the list of the .wav files that were in the folder you specified in the steps above.
Since Praat scripts can only operate with things that are in its own world, we needed to create this list of elements to the Objects window when we are scripting.Back to the script. Line 3 creates a numeric variable with a value of 3. Line 4 recovers the string number 3 from the Strings list, i.e. carta.wav, through the command Get string, and stores it as a string variable called thisfile$. The Get string command takes a number as an argument, and the number refers to the elements in the Strings list. Line 5 reads the file and takes it to the Objects window; finally, line 6 plays it.
Did the script run? If so, you should have been able to hear file number 3, i.e. carta.wav. If you get an error message, make sure that the path to your folder is correct, and that there are no typos in your script (mind the capitals and commas!).
IMPORTANT: You can save your scripts by going to File >> Save as. Name your script and make sure that the extension is .praat. After this you will be able to open your script with Praat and run it again.
3 Homework
As a homework, you will have to:
- Open a new Praat script.
- Create a small script that opens and plays the sound melon.wav (note: you don't need to create a Strings list for this). Make it print an info line that gives you the name of the file being opened, e.g. something like "Opening sound melon.wav" as the script runs. Pro tip: Check the Praat manual for string functions (Help >> Search Praat manual)
- Save your script as hw1_yourlastname.praat and upload it to the Assignments folder on ILIAS. You won't be graded for this, but it counts as a prerequisite to pass the course and it will give me the opportunity to provide feedback on your work.
- Now, go to Edit >> Clear history (in the script window). Then, open and play melon.wav manually. Go back to the Praat script, and go to Edit >> Paste history. What did you get?